What and How to Use an API Key: Your Guide to Secure Integration
An API key is a secure method used to authenticate a user, application, or device making a request to an API (Application Programming Interface). It’s a unique identifier, similar to a password, that allows access to a service’s API and is used to track how the API is being utilized. Unlike user credentials that are designed for personal use, an API key is specifically meant for software access.
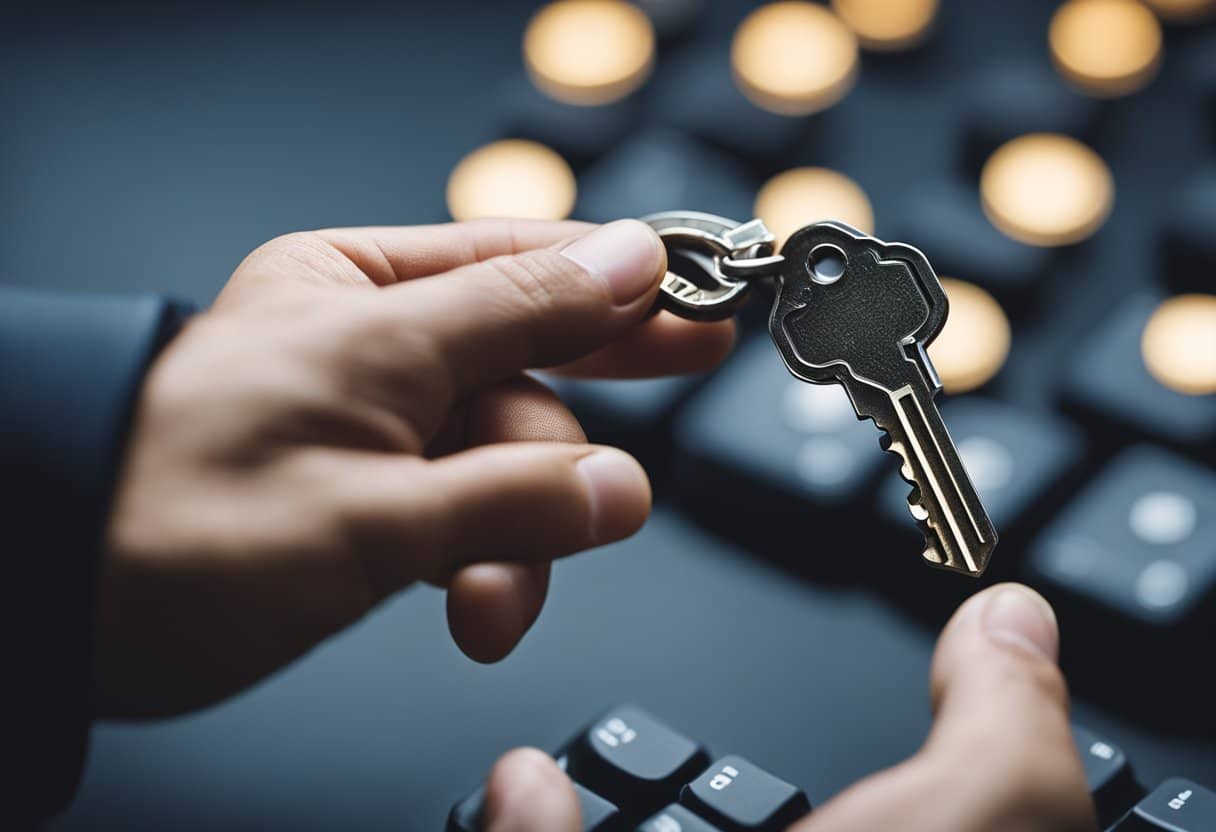
API keys are essential for controlling the usage of APIs and ensuring that the data exchanged remains secure. When a developer integrates an API into their application, using the API key tells the server which application is making the request. This process of authentication helps providers keep track of API usage by different applications and prevent misuse by unauthorized parties.
Key Takeaways
- An API key is a unique identifier used to authenticate and track API usage.
- It is crucial for securing API interactions between applications and services.
- Proper management and integration of API keys ensure secure and efficient use of APIs.
Understanding API Keys
API keys serve as both an identifier and a security mechanism to control access to APIs. They come in various forms, each with specific purposes and security measures to protect sensitive data.
Definition and Purpose
An API Key is a unique identifier that authenticates requests to an API, ensuring that the caller has permission. It’s like a digital passcode that allows software applications to communicate with each other securely. These keys help prevent unauthorized access and are crucial for API Key Management, which involves keeping track of keys and ensuring they’re not misused.
- Public API Keys are meant for less sensitive operations that don’t require user-specific data.
- Private API Keys are used for more sensitive operations and should be kept confidential to protect sensitive data.
Different Types of API Keys
There isn’t just one type of API key; each serves a different purpose:
- Simple API Keys: These are passed in the HTTP request—either in the header (
X-API-Key
) or as a query parameter. - Bearer Tokens: Unlike simple keys, tokens often carry more information and represent the authenticated user, not just the application.
Proper usage of API keys involves implementing the correct type based on the application’s needs.
Security Considerations
Protecting Sensitive Data is paramount when using API keys. These measures help maintain the integrity and confidentiality of the API:
- Encryption: Always transfer API keys over SSL/TLS channels.
- Access Limits: Apply strict permissions for keys to limit access to data.
- Rotation and Expiration: Regularly change API keys to minimize the risk of compromise.
Understanding and implementing these security considerations is crucial for maintaining a secure API ecosystem.
API Key Authentication
API key authentication is a simple yet effective way to control access to an API. It utilizes a unique key to verify a user or application’s identity, ensuring secure interactions with the API.
Mechanisms of Authentication
The basic mechanism involves sending a unique key with each API request, which the server checks against a list of authorized keys. Authentication can be done via the query string, by including the API key as a parameter in the URL, or through request headers. The header method is often considered more secure than the query string, as URLs can be cached or logged, potentially exposing the API key.
Example of header authentication:
GET /api/resource HTTP/1.1
Host: example.com
x-api-key: YOUR_API_KEY
While API keys can provide a first level of security, they are sometimes used in combination with other methods for enhanced security. OAuth is an open-standard authorization protocol or framework that describes how unrelated servers and services can safely allow authenticated access to their assets without actually sharing the initial, related, single logon credential. JWT (JSON Web Tokens) is another method, which securely transmits information between parties as a JSON object. When it comes to access control, API Key Authentication is a method that can be effectively combined with these protocols to determine what resources a user can access.
Best Practices for Secure Authentication
Secure usage of API keys demands adherence to best practices:
- Do not embed API keys directly in code: Instead, use environment variables or configuration files.
- Restrict API key permissions: Assign only the necessary permissions for a specific task to minimize risks.
- Rotate API keys regularly: Changing API keys periodically limits the risk if they get compromised.
- Monitor usage: Keep an eye on how and when the API keys are used to detect unauthorized access.
- Use HTTPS: Encrypting transmissions via HTTPS prevents the API key from being intercepted during transit.
For instance, while authenticating APIs, methods such as x-api-key, Basic Auth, and Bearer Auth have different implications for security and usability. Users should learn how to use API keys to authenticate APIs with these methods, balancing the pros and cons of each approach. In addition, understanding what API authentication is, its benefits, and methods proves crucial in choosing the most fitting authentication strategy for their API.
Making API Requests
When working with APIs, developers initiate communication using specific request methods, address the correct endpoints, and then properly handle the response data. Each of these steps is crucial for the successful integration and use of an API.
Common Request Methods
In API communication, there are several HTTP request methods that clients use to interact with resources. The GET request is used to retrieve data from an API, sending back the requested information. A POST request sends data to the API for a new resource creation, while PUT updates an existing resource. DELETE is self-explanatory; it removes the specified resource.
- GET: Retrieve data.
- POST: Create a new resource.
- PUT: Update an existing resource.
- DELETE: Remove a resource.
Understanding Endpoints
Endpoints refer to specific paths defined by an API where requests are sent. They are the points of entry for an API request and dictate the types of operations that can be performed. An endpoint for a GET request might return user data, while a POST request endpoint could be used to create a new user profile.
- Format:
<protocol>://<hostname>/<resource>
- Example:
https://api.example.com/users
Handling Responses
After an API request is made, an API response is returned which includes a status code indicating the success or failure of the request. Common status codes are:
200
: Success — Request has succeeded.201
: Created — The request has led to a new resource being created.400
: Bad Request — The server cannot process the request due to client error.401
: Unauthorized — Authentication is required and has failed or has not been provided.404
: Not Found — The requested resource could not be found.
Responses often come in a structured format like JSON, containing the data requested or a message detailing the result of the API call.
API Request Tools and Platforms
There are several tools and software that streamline the process of making API calls and managing API interactions. These include user-friendly applications and robust online platforms that provide developers with the necessary functionalities to test, document, and secure their API endpoints.
Using Postman for API Calls
Postman is a popular tool among developers for testing RESTful APIs. Through its intuitive interface, it allows for the creation, sharing, testing, and documentation of APIs. The users can organize API requests into collections and folders for better management. Postman also supports various methods of API authentication, allowing developers to include their API keys securely. It’s a versatile environment for both building and consuming APIs.
- Creating Requests: Users can input their API server’s endpoint URL and select the appropriate HTTP method.
- Adding Headers/Params: Postman allows users to add necessary headers, including API keys for authentication.
- Executing Calls: With just a click of a button, Postman sends the request to the API server and displays the response.
Leveraging Platforms like RapidAPI
RapidAPI is a comprehensive platform that connects developers to thousands of public APIs in one place. The platform simplifies the integration of various APIs into software development projects. It provides a unified interface for executing API calls, with features that support discovering, testing, and managing APIs. Additionally, RapidAPI’s marketplace offers features like interactive API documentation to help understand how to make API requests effectively.
- API Marketplace: Developers can browse through a vast collection of APIs in various categories.
- Interactive Documentation: Offers clear descriptions and usage examples for API endpoints.
- API Management: Tools for monitoring usage, access control, and analytics are available on the platform.
Integrating APIs
When incorporating an API into software development, one must adhere to best practices for design and documentation while being prepared to address issues that may arise. This ensures that the integration process is smooth and that the resulting application functions reliably.
API Integration Best Practices
In the realm of API integration, best practices revolve around thorough understanding and meticulous planning. A developer should always refer to the API documentation which typically outlines the API Specification. This helps in understanding what the API endpoints can do.
- Use Proper Authentication: Secure API keys properly, as explained in guides like What Is an API Key?, to ensure that requests are authenticated.
- Follow the API Design: Adhere strictly to the API’s design principles to avoid unexpected responses or errors.
- Version Control: Make use of the versioning information provided to ensure compatibility with the API.
Testing plays a critical role in ensuring the reliability of an API integration. Developers should:
- Create various test cases to cover possible scenarios.
- Use a staging environment to simulate real-world use before going live.
Troubleshooting Common Integration Issues
Even with careful planning, issues can arise. Knowing common troubleshooting steps is vital.
- Examine Network Calls: Monitoring tools like Network tab in browser DevTools can help identify the status of API requests and responses.
- Read Error Messages: They often provide clues that can guide one towards the source of the problem.
- Check the API Endpoint: Ensuring the correct endpoint is targeted is crucial, as a misconfigured endpoint can lead to failures.
Logs are also valuable; they should be checked regularly to spot and understand issues early. If the problem persists, developers should check the API documentation again, as it might offer insights into limitations or changes in the API that they weren’t aware of.
API Design and Documentation
Effective API design ensures that a service can be used by a wide range of clients, whereas comprehensive API documentation provides the necessary instructions and guidelines for developers to integrate effectively with the API.
Creating a Well-Defined API
Designing an API involves making deliberate choices about exposing data and functionality to users in a usable way. A well-defined API follows certain principles to meet the needs of its users, whether they are Open APIs, available to external developers, Partner APIs, restricted to business partners, or Internal APIs, used within an organization.
API Specification: Clear and standardized specifications are the foundation of good API design. Specifications like OpenAPI define the structure of an API, including endpoints, methods, parameters, and data formats. These specifications serve as a contract that ensures consistency and reliability.
- Endpoints: Define access points for different functions.
- Methods: GET, POST, DELETE, etc., explain operations performed at each endpoint.
- Parameters: Query strings, headers, and body content that clients must provide.
- Data formats: JSON, XML for request and response body content.
For a successful API, one must design keeping in mind the future scalability and potential for integration with other systems, often resulting in Composite APIs that combine various services and data sources.
Documenting APIs for Better Usability
Documentation is crucial for any API’s usability. It provides a set of instructions and examples that help developers understand and integrate the API into their projects. Good documentation enhances the developer experience and can reduce integration time significantly.
Key aspects of API Documentation:
- Human-readable instructions: Clearly describe how to use the API, with examples.
- Authentication protocols: Explain how to obtain and use an API key effectively.
- Common requests and responses: Illustrate with real-world examples.
- Errors: Define common error codes and messages to help troubleshoot issues.
Tools like Postman and Swagger offer solutions for creating and maintaining accurate API documentation, often auto-generating this documentation from the API design and specification. These tools help in visualizing and interacting with the API, which is a significant aid in both designing APIs and creating documentation that accurately communicates the API’s capabilities and limitations.
Managing API Keys
Proper management of API keys is critical to maintain security and control over the services that these keys access. This includes ensuring secure storage with limited access, and monitoring their use to prevent abuse.
Secure Storage and Rotation
Securing API keys involves keeping them out of public view and restricting access to only those who legitimately need them. Best practices suggest storing keys in secure environment variables or dedicated secrets management systems, which prevents accidental leaks through source code.
API keys are sensitive, and periodic rotation is a critical step to guard against unauthorized use. One can start by listing all the keys used in their systems, noting down where they are located, and then establish a routine in which these keys are changed at regular intervals, rendering the old key useless and replacing it with a new one.
- Storage options:
- Environment variables
- Dedicated secret management service
- Rotation frequency:
- Based on risk assessment
- Regular schedule (e.g., every 90 days)
Monitoring and Throttling Usage
It’s important for one to monitor the number of calls made with their API keys to detect any unusual patterns which might indicate a breach or misuse. Many platforms provide built-in tools to track the history of API key requests, making it easy for one to observe the volume of traffic and the corresponding response statuses.
Throttling is a technique used to limit the number of calls an API key can make in a given time period. By setting these limits, one can prevent abuse and ensure fair usage among all users.
- Monitoring Strategies:
- Review logs for unusual activity
- Set up alerts for anomalies
- Throttling Methods:
- Rate limit per key
- Quotas based on time interval
By putting in place secure storage options, a scheduled rotation policy, and effective monitoring, together with the application of throttling, API key management becomes a robust defense against the potential misuse of keys.
Types of APIs

APIs come in different forms, each designed to serve a unique purpose and audience. They vary based on their accessibility and the scope of functionality they offer.
Open vs Private APIs
An Open API, sometimes referred to as a Public API, is typically designed for external use. They are available to developers and other users with minimal restrictions. One might need to register or acquire an API key, but the goal is for wide-reaching compatibility and use.
On the other hand, a Private API is intended for use within the organization that created it. These are not available for public use and are designed to improve internal development and processes.
Internal, Partner, and Composite APIs
Internal APIs are a subset of private APIs which are used within an organization. They help streamline operations by enabling different internal systems to communicate with each other.
Partner APIs are designed to be shared outside of the company but are limited to specific business partners. These APIs facilitate cooperation and are a key component of business strategies that involve close integration with external systems.
Composite APIs combine different data and service APIs. They are a sequence of tasks that run synchronously as a result of the execution and not necessarily the request of an end-user. They provide a more streamlined way to handle multiple tasks, like viewing a page with multiple components.
API Key Usage Examples
API keys are essential for accessing certain web services that require authentication. They are a simple way to help keep the connection between a user’s request and the response from an API safe and secure.
Example: Google Maps API
A common use of an API key is with the Google Maps API, where it is employed for map-related services. For instance, if someone needs to embed a Google Map on their website, they will need a Google Maps API key. This unique key is included in the web page’s code to confirm the user’s identity and to track API usage.
To get the API key for Google Maps, one must create a project within the Google Cloud Platform. Once the key is acquired, it’s integrated into the application by adding it to the request URL like so:
https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap
It’s crucial to keep this key private, as unauthorized access could lead to misuse, potentially resulting in extra charges if usage limits are exceeded.
Example: Custom API Integration
For custom API integration, using an API key differs slightly but follows a similar security principle. When a developer builds a custom application that must interact with an external service, an API key is often used to manage and allow secure access.
For example, if a developer is working on a weather application, they might use an external weather service API to fetch weather data. The API key is included in the header of the HTTP request, like this:
GET /api/weather/today HTTP/1.1
Host: example-weather-service.com
X-API-Key: abc123456789
In this example, X-API-Key
is a custom header used for passing the API key. Custom APIs might have different header names for API keys, but the concept remains constant across various services – it’s a unique identifier that authenticates and authorizes the API request.
By properly using API keys in these contexts, not only is security maintained but developers can track and limit usage as per the requirements of the service provider.
Frequently Asked Questions

Navigating the integration, generation, locating, and securing of API keys is essential for developers and businesses. Correct usage and security practices ensure the smooth operation of digital services.
How does one integrate an API key into a website?
Integrating an API key into a website involves adding the key to API requests for authentication purposes. It typically resides in the header of an HTTP request or as part of the URL as a query parameter.
What are the steps to generate an API key?
Generating an API key usually involves creating an account with the service offering the API and navigating their dashboard to access the API management section where keys can be created.
How can one find their API key for a service?
One can find their API key for a service by logging into the service’s user account and looking for sections like “API,” “Credentials,” or “Security” within the account or settings menus.
In which scenarios should one use a sample API key?
A sample API key can be used while exploring the capabilities of an API in a controlled environment, such as a sandbox or a documentation explorer, before using a personal, private key.
What measures should one take to secure their API key?
To secure an API key, one should keep it confidential, restrict access to the key, regularly rotate the key, and monitor usage to detect any unauthorized or abnormal activity.
How do API keys enhance the security of applications?
API keys act as a unique identifier that verifies the identity of the requester, limiting and controlling access to API functions and protecting against unauthorized use.